Utility helpers for Enums
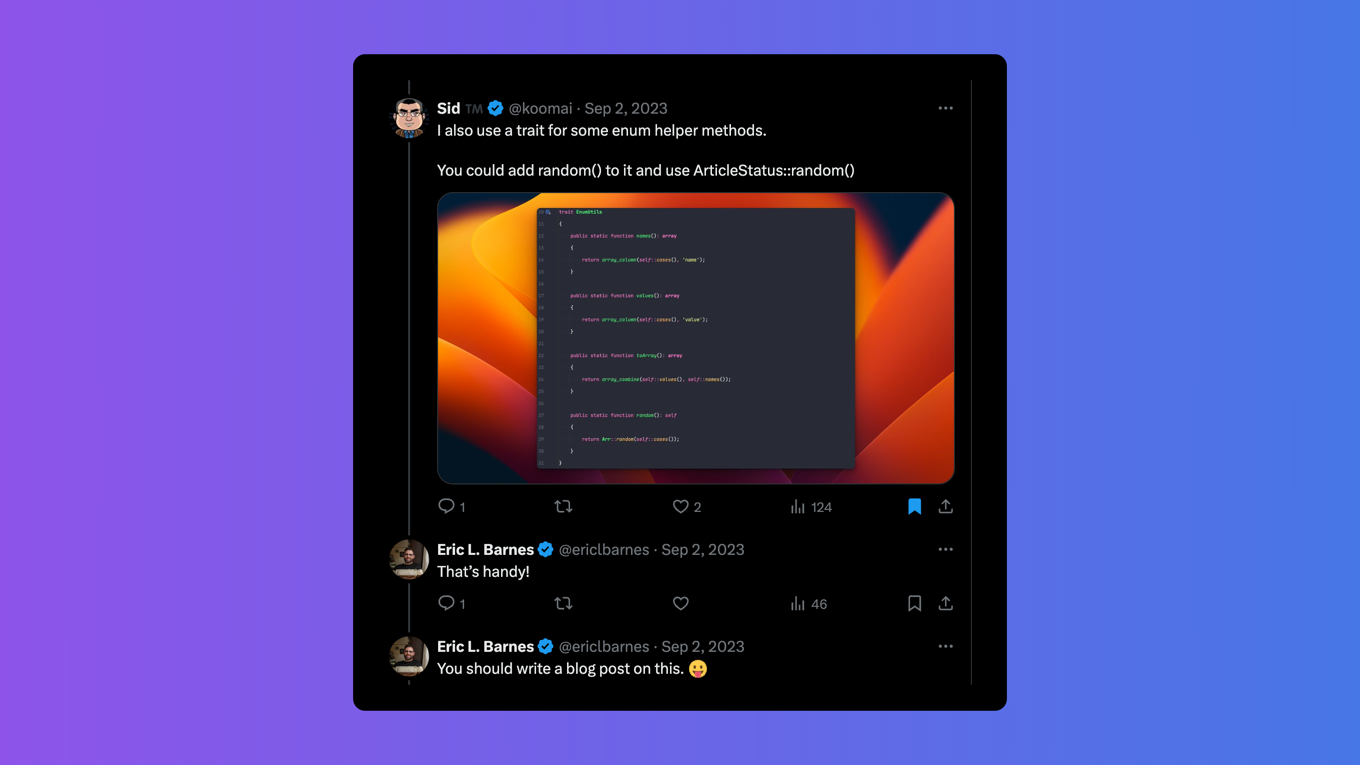
PHP has had native Enumerations (Enums) since version 8.1 was released. However, they lack some convenience methods that I need for some operations in a project I'm working on.
We'll look at how I use a trait to add some functionality to my enum classes.
This is my HasEnumUtils
trait:
<?php
namespace App\Enums;
use Illuminate\Support\Arr;
trait HasEnumUtils
{
public static function names(): array
{
return array_column(self::cases(), 'name');
}
public static function values(): array
{
return array_column(self::cases(), 'value');
}
public static function toArray(): array
{
return array_combine(self::names(), self::values());
}
public static function random(): self
{
return Arr::random(self::cases());
}
}
We include it as usual in an enum class that needs the additional functionality:
enum UserRole: string
{
use HasEnumUtils;
case ADMIN = 'admin';
case EDITOR = 'editor';
case MODERATOR = 'moderator';
case READER = 'reader';
}
Usage
$allowedRoles = UserRole::names();
// ['ADMIN', 'EDITOR', 'MODERATOR', 'READER']
The names()
method returns an array of all the enum case names. We're using a Backed Enum as an example but this is more useful for Pure Enums where there are no corresponding string values for cases.
$allowedRoles = UserRole::values();
// ['admin', 'editor', 'moderator', 'reader']
Similar to names()
, the values()
method returns an array of all the enum case values. This is handy when building options for a <select>
tag in the UI, or the select function in Prompts.
You can also use it in your validation rules:
$request->validate([
'role' => ['required', Rule::in(UserRole::values())],
]);
$roleMap = UserRole::toArray();
// [
// 'ADMIN' => 'admin',
// 'EDITOR' => 'editor',
// 'MODERATOR' => 'moderator',
// 'READER' => 'reader'
//]
The toArray()
method combines the names and values into an associative array. This is useful if you want to create an associative array of case/value for an array map.
[
'ADMIN' => 'admin',
// ...
]
vs
[
App\Enums\UserRole {#6432
+name: "ADMIN",
+value: "admin",
},
// ...
]
$randomRole = UserRole::random();
// UserRole::EDITOR
random()
returns a random case. This is useful for generating random values in Eloquent factories.
public function definition()
{
return [
'name' => fake()->name(),
'email' => fake()->unique()->safeEmail(),
'role' => UserRole::random(),
// other fields...
];
}
Liked this article? Share it on X